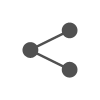
Seraphinite Accelerator is a powerful tool that optimizes the speed of WordPress websites and manages caching. In this article, we will cover how to delete the generated cache using the API.
At COOSS.NET, when writing articles in Korean, we have created a function in the theme’s functions.php that automatically performs translations, and on translated pages, links to select other languages are generated. However, there was an issue where, after the initial article is cached by the Accelerator, even if there are translated pages, the link to the translated page does not appear on the cached page.
Of course, it is possible to set the Accelerator to periodically refresh the cache, but it is cumbersome to change the settings every time just to refresh the translated pages. Moreover, forcing a complete site cache refresh is not efficient, so we looked for an API that would force the original page cache to be regenerated once the translation is complete.
Seraphinite Accelerator API Overview
Seraphinite Accelerator provides the following API functionalities.
OperateCache – Cache Management
You can delete or regenerate the cache of a specific page.
GetCacheStatus – Check Cache Status
You can check whether a specific URL is cached.
Cache Management API (OperateCache)
The OperateCache function can delete or regenerate the cache of a specific URL as needed.
Usage
seraph_accelAPI::OperateCache( $op, $obj );
Parameter Description
- $op (Cache management option)
- CACHE_OP_REVALIDATE → Cache revalidation
- CACHE_OP_CHECK_REVALIDATE → Revalidate cache if necessary
- CACHE_OP_CLEAR → Clean up old cache
- CACHE_OP_DEL → Delete cache
- CACHE_OP_SRVDEL → Delete server cache (if possible)
- $obj (URL or relative path to delete)
- Enter the URL to delete the cache of a specific page
- Enter null to delete the entire site cache
Example of Cache Deletion
Delete Cache of Specific Page
seraph_accelAPI::OperateCache( seraph_accelAPI::CACHE_OP_DEL, '/mypage/' );
Delete Entire Site Cache
seraph_accelAPI::OperateCache( seraph_accelAPI::CACHE_OP_DEL, null );
Delete Cache of Multiple URLs
$urls = ['/category/wordpress/', '/tag/performance/', '/custom-post-type/my-post/'];
foreach ($urls as $url) {
seraph_accelAPI::OperateCache( seraph_accelAPI::CACHE_OP_DEL, $url );
}
Cache Status Check API (GetCacheStatus)
You can check whether a specific URL is cached and whether cache optimization has been applied.
Usage
$cache_status = seraph_accelAPI::GetCacheStatus($obj, $headers = []);
Parameter Description
- $obj → URL to check
- $headers (optional) → Pass specific HTTP headers to check the cache
- Example: ‘User-Agent’ => ‘AppleWebKit/9999999.99 Mobile’
Return Values
Property | Value | Meaning |
---|---|---|
cache | true | The page is cached |
cache | false | The page is not cached |
optimization | true | Cache optimization completed |
optimization | false | Cache not optimized |
optimization | null | No cache |
status | 'done' | Caching completed |
status | 'revalidating' | Cache regeneration in progress |
status | 'pending' | Cache pending |
status | 'none' | Not processed |
Example of Cache Status Check
$cache_status = seraph_accelAPI::GetCacheStatus('https://example.com/mypage/');
if ($cache_status['cache']) {
echo 'The page has been cached.';
} else {
echo 'The page has not been cached.';
}
Creating a Function to Delete Cache for a List of Specific URLs
You can create a function to delete the cache of multiple URLs at once.
Cache Deletion Function
function sa_clear_cache_for_urls($urls = []) {
if (!class_exists('seraph_accelAPI')) {
error_log('Seraphinite Accelerator API is not loaded.');
return false;
}
if (!is_array($urls) || empty($urls)) {
error_log('A valid list of URLs was not provided.');
return false;
}
foreach ($urls as $url) {
if (!empty($url)) {
seraph_accelAPI::OperateCache(seraph_accelAPI::CACHE_OP_DEL, $url);
error_log('Cache deleted: ' . $url);
}
}
return true;
}
Usage Example
$urls_to_clear = [
'/category/wordpress/',
'/tag/performance/',
'/custom-post-type/my-post/',
'/',
];
sa_clear_cache_for_urls($urls_to_clear);
By defining the function as above, you can pass the original article page address to the function at the point when the translation is complete to refresh the cached content (after deletion, the page will be refreshed at the time it is displayed or as set in the Accelerator settings).