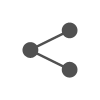
Have you heard of 301 redirect? If you’re running a WordPress site, you can implement it using a shortcode, and I would like to outline how to do this using the 301 Redirect Shortcode.
What is a 301 Redirect Shortcode?
While operating a website, there may be times when a user tries to access a specific page that no longer exists. From a search engine’s perspective, if this happens frequently, it may lower the site’s credibility.
In such cases, using a 301 redirection can automatically guide visitors to another page. A 301 redirection informs visitors (and search engines) that the content they are trying to access has been moved to a different URL and connects them to the new URL page. This improves user experience and can also aid in SEO Optimization.
Why is a 301 Redirect Shortcode Necessary?
Let’s take a deeper look at the necessity of the 301 redirect shortcode. Website operators set up redirections for the following reasons:
- Page Deletion: When a page that is no longer in use is deleted, it can guide users to the homepage or another useful page.
- URL Change: When the website structure or URL is changed, it allows automatic redirection to the new URL even if the old URL is accessed.
- SEO Optimization: It prevents 404 errors that can occur when users try to find non-existent pages, thereby enhancing the site’s credibility and improving SEO scores.
How to Write a 301 Redirect Shortcode
Now, let’s write a shortcode that can implement a 301 redirection. Below is an example of that code:
add_action( 'template_redirect', function () {
$request_uri = parse_url( $_SERVER['REQUEST_URI'], PHP_URL_PATH );
$request_uri = trim( $request_uri, '/' );
$blocked_paths = [
'login',
'sample-page',
];
if ( in_array( $request_uri, $blocked_paths, true ) ) {
wp_redirect( home_url( '/' ), 301 );
exit;
}
} );
Explaining the Code
This code is quite simple. It checks the requested URI by the user, and if that URI matches one of the blocked paths (recorded in $blocked_paths), it redirects the user to the homepage with a 301 redirection. It’s good practice to comment on the paths added to the ‘blocked_paths’ variable for easier management later.
template_redirect
The function starts with add_action( ‘template_redirect‘, function () {..}.
template_redirect
is an action hook that runs just before WordPress loads the template file. ‘Action hooks’ are hidden throughout the WordPress source code for extensibility, allowing developers to add necessary features to their websites.- In other words, it occurs right before WordPress decides which template (
single.php
,page.php
,archive.php
,404.php
, etc.) to load when a user accesses a URL. - This means that the function written in function() { … } will be executed at this point.
- The timing of when the template_redirect action hook executes in the list of functions WordPress performs internally to open a page can be displayed as follows.
index.php
-> wp-blog-header.php
-> wp-load.php
-> wp-config.php
...
-> wp()
-> $wp->main()
-> parse_request()
-> query_posts()
-> handle_404()
-> template_redirect() //← Right here!
-> load_template()
You can also separate the callback function used in add_action() and write the code as follows.
function my_custom_redirect_logic() {
// Redirection logic
}
add_action( 'template_redirect', 'my_custom_redirect_logic' );
Function Details
- It retrieves the requested path from
$_SERVER['REQUEST_URI']
, removes the slashes (/
), and checks it. - If a user attempts to access pages like ‘login’ or ‘sample-page’, this shortcode automatically redirects them to the homepage. This provides a better experience for users and increases the utility of the website.
- The
template_redirect
hook operates just before loading the theme template, making it suitable for intercepting page processing.
Usage
- Add the above code directly into the functions.php file located in your currently used theme folder.
- Change or add the ‘login’ and ‘sample-page’ parts to the actual URLs you need.
Notes
- If you want to handle URLs without case sensitivity, you can use
strtolower()
. - It ignores query strings and recognizes only the path (e.g.,
/login?redirect=true
→/
). - You can also use
wp_safe_redirect()
, but it has restrictions that only allow redirection to the same domain, sowp_redirect()
is more suitable here. wp_redirect()
is the basic function provided by WordPress for executing redirections.
Real Use Cases
There are various situations where you can utilize this 301 Redirect Shortcode. For instance, sometimes you may renew your website or discontinue a specific service, making previously used pages unavailable. By setting up a 301 redirection in such cases, users can click on an incorrect page without getting lost and can still find other useful information.
In Conclusion
The 301 Redirect Shortcode plays an important role in enhancing user experience and positively impacting SEO. Website managers can systematically manage pages and avoid unnecessary 404 errors through these shortcodes, so I highly recommend utilizing them.
If you are using an SEO plugin like Rank Math, you can also utilize it. (I will organize related content separately)
[SEO] : SEO Optimization, the process of increasing a website’s visibility in search engines.
[wp_redirect] : A WordPress function that guides users to a specific URL and handles redirection.